Going back to Squareline Studio (SLS), I incorporated the TouchScreen library and code from this previous experiment. I added a, very very simple, second screen:
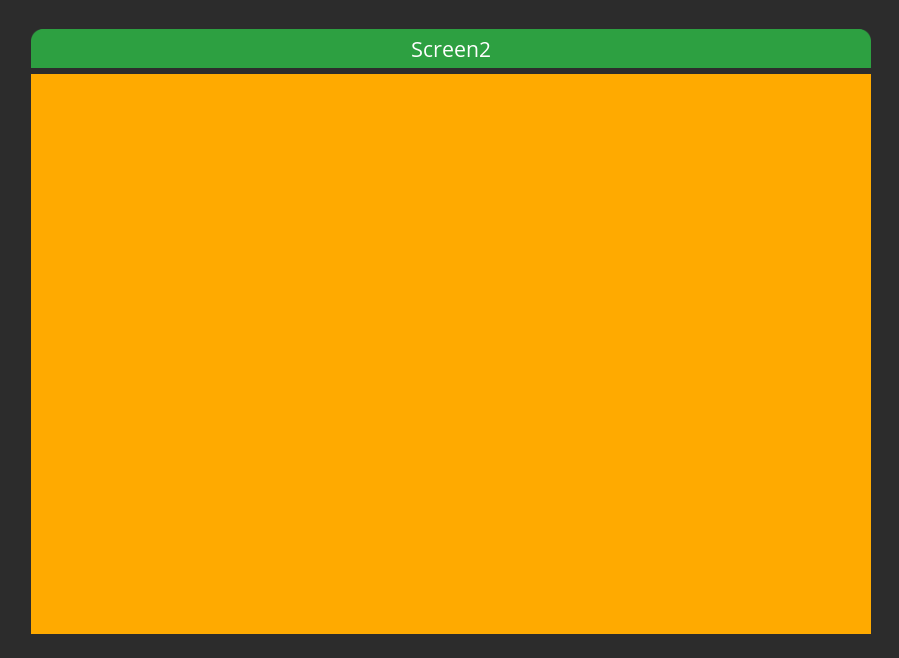
Then added an Event to each screen with the “Released” trigger:
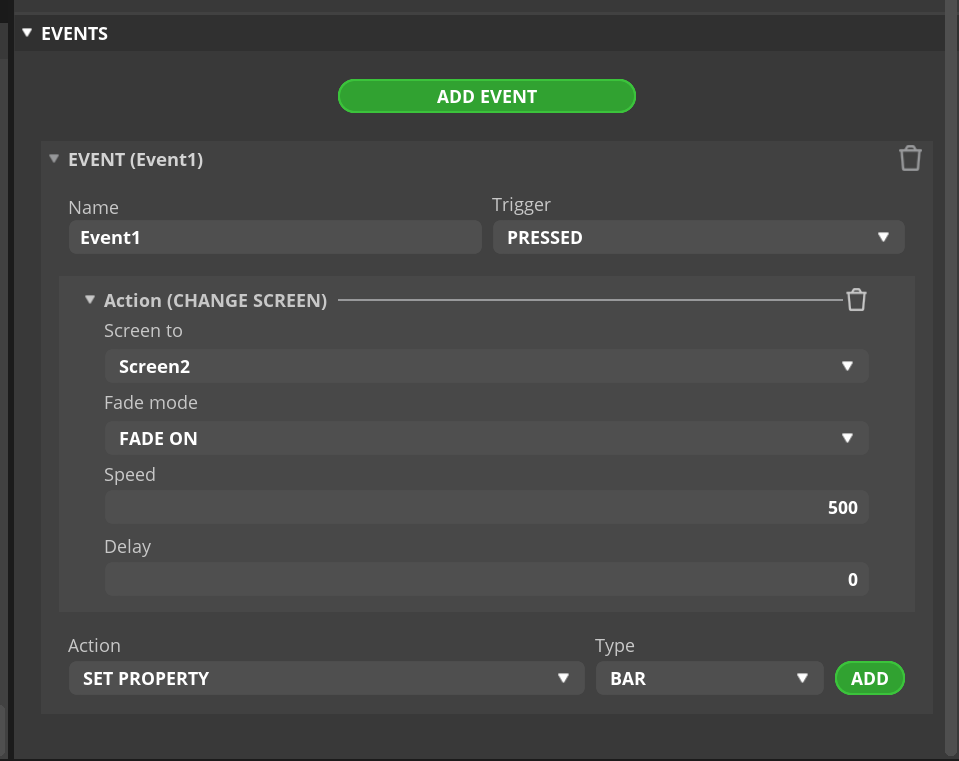
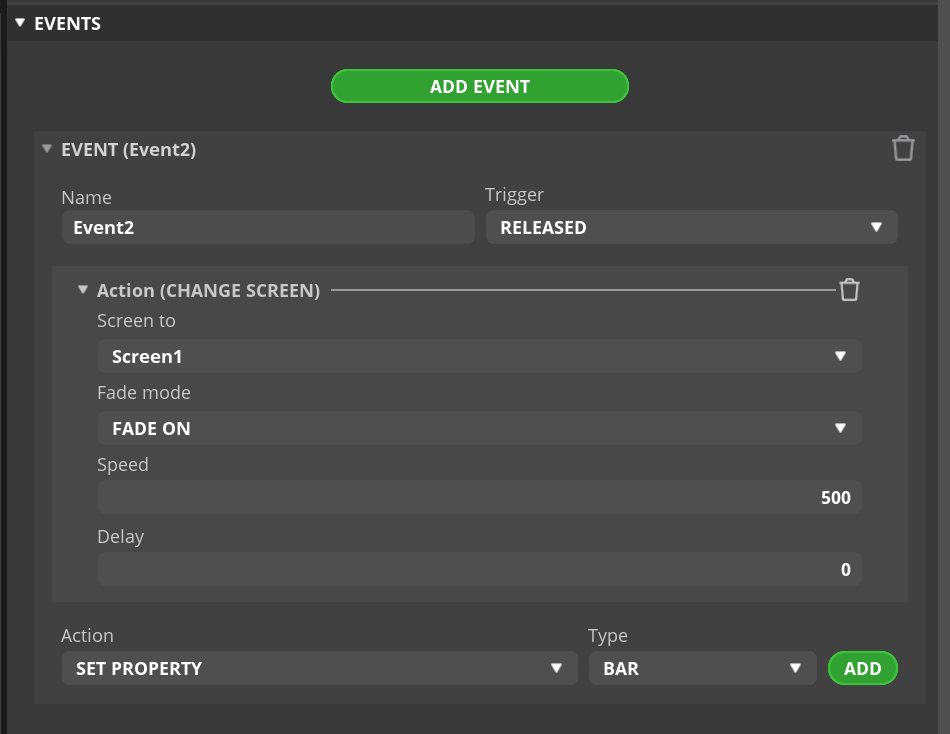
Here’s the complete code, interesting bits are bolded:
//Arduino-TFT_eSPI board-template main routine. There's a TFT_eSPI create+flush driver already in LVGL-9.1 but we create our own here for more control (like e.g. 16-bit color swap).
#include <lvgl.h>
#include <TFT_eSPI.h>
#include <ui.h>
#include <TouchScreen.h>
/*Don't forget to set Sketchbook location in File/Preferences to the path of your UI project (the parent foder of this INO file)*/
/*Change to your screen resolution*/
static const uint16_t screenWidth = 480;
static const uint16_t screenHeight = 320;
enum { SCREENBUFFER_SIZE_PIXELS = screenWidth * screenHeight / 10 };
static lv_color_t buf [SCREENBUFFER_SIZE_PIXELS];
TFT_eSPI tft = TFT_eSPI( screenWidth, screenHeight ); /* TFT instance */
#define YP A19 // must be an analog pin, use "An" notation!
#define XM A15 // must be an analog pin, use "An" notation!
#define YM 14 // can be a digital pin
#define XP 27 // can be a digital pin
// Touchscreen coordinates: (x, y) and pressure (z)
// For better pressure precision, we need to know the resistance
// between X+ and X- Use any multimeter to read it
// For the one we're using, its 281 ohms across the X plate
TouchScreen ts = TouchScreen(XP, YP, XM, YM, 281);
int x, y, z;
#if LV_USE_LOG != 0
/* Serial debugging */
void my_print(const char * buf)
{
Serial.printf(buf);
Serial.flush();
}
#endif
/* Display flushing */
void my_disp_flush (lv_display_t *disp, const lv_area_t *area, uint8_t *pixelmap)
{
uint32_t w = ( area->x2 - area->x1 + 1 );
uint32_t h = ( area->y2 - area->y1 + 1 );
if (LV_COLOR_16_SWAP) {
size_t len = lv_area_get_size( area );
lv_draw_sw_rgb565_swap( pixelmap, len );
}
tft.startWrite();
tft.setAddrWindow( area->x1, area->y1, w, h );
tft.pushColors( (uint16_t*) pixelmap, w * h, true );
tft.endWrite();
lv_disp_flush_ready( disp );
}
/*Read the touchpad*/
void my_touchpad_read (lv_indev_t * indev_driver, lv_indev_data_t * data)
{
uint16_t touchX = 0, touchY = 0, touchZ = 0;
// Get Touchscreen points
TSPoint p = ts.getPoint();
// Checks if Touchscreen was touched, and prints X, Y and Pressure (Z)
if ( p.z > 15 ) {
// Calibrate Touchscreen points with map function to the correct width and height
touchX = map(p.y, 970, 205, 1, screenWidth);
touchY = map(p.x, 248, 875, 1, screenHeight);
touchZ = p.z;
data->state = LV_INDEV_STATE_PR;
// Set the coordinates
data->point.x = touchX;
data->point.y = touchY;
Serial.print( "Data x " );
Serial.println( touchX );
Serial.print( "Data y " );
Serial.println( touchY );
Serial.print( "Data z " );
Serial.println( touchZ );
}
else
{
data->state = LV_INDEV_STATE_REL;
}
}
/*Set tick routine needed for LVGL internal timings*/
static uint32_t my_tick_get_cb (void) { return millis(); }
void setup ()
{
Serial.begin( 115200 ); /* prepare for possible serial debug */
// Set ADC resolution required for TouchScreen library
analogReadResolution(10);
String LVGL_Arduino = "Hello Arduino! ";
LVGL_Arduino += String('V') + lv_version_major() + "." + lv_version_minor() + "." + lv_version_patch();
Serial.println( LVGL_Arduino );
Serial.println( "I am LVGL_Arduino" );
lv_init();
#if LV_USE_LOG != 0
lv_log_register_print_cb( my_print ); /* register print function for debugging */
#endif
tft.begin(); /* TFT init */
tft.setRotation( 3 ); /* Landscape orientation, flipped */
static lv_disp_t* disp;
disp = lv_display_create( screenWidth, screenHeight );
lv_display_set_buffers( disp, buf, NULL, SCREENBUFFER_SIZE_PIXELS * sizeof(lv_color_t), LV_DISPLAY_RENDER_MODE_PARTIAL );
lv_display_set_flush_cb( disp, my_disp_flush );
static lv_indev_t* indev;
indev = lv_indev_create();
lv_indev_set_type( indev, LV_INDEV_TYPE_POINTER );
lv_indev_set_read_cb( indev, my_touchpad_read );
lv_tick_set_cb( my_tick_get_cb );
ui_init();
Serial.println( "Setup done" );
}
void loop ()
{
lv_timer_handler(); /* let the GUI do its work */
delay(5);
}